Create plugin#
How to create new plugin#
To create a plugin, you will need a bit of knowledge about PHP language programming and OOP (object-oriented programming). The plugin interface is simple and it does not require knowing how Bacularis works internally.
Notification plugin example#
In the Bacularis Web project directory is a new directory named Plugins
:
[main project dir]/protected/Web/Plugins/
If you have installed Bacularis using binary packages, the path can be the following for the Bacularis Web plugins:
/usr/share/bacularis/protected/Web/Plugins/
In the Plugins
directory please create a new PHP file that will contain
the plugin code. Exmaple:
/usr/share/bacularis/protected/Web/Plugins/ExamplePlugin.php
It will contain a PHP class that needs to implement an interface according to
desired plugin type. In this example we use the notification plugin interface
IBacularisNotificationPlugin
. The plugin class should extend the plugin
base class BacularisWebPluginBase
.
<?php
use Bacularis\Common\Modules\IBacularisNotificationPlugin;
use Bacularis\Web\Modules\BacularisWebPluginBase;
class ExamplePlugin extends BacularisWebPluginBase implements IBacularisNotificationPlugin
{
/**
* Get plugin name displayed on the web interface.
*
* @return string plugin name
*/
public static function getName(): string
{
return 'My example plugin';
}
/**
* Get plugin version.
*
* @return string plugin version
*/
public static function getVersion(): string
{
return '1.0.0';
}
/**
* Get plugin type.
* For notification plugins it should be 'notification'.
*
* @return string plugin type
*/
public static function getType(): string
{
return 'notification';
}
/**
* Get plugin configuration parameters.
*
* return array plugin parameters
*/
public static function getParameters(): array
{
return [
['name' => 'myparameter1', 'type' => 'string', 'default' => 'Def. Value 123', 'label' => 'My parameter 1'],
['name' => 'myparameter2long', 'type' => 'string_long', 'default' => "One\nTwo\nThree", 'label' => 'My multi-line string'],
['name' => 'mynumber', 'type' => 'integer', 'default' => 10, 'label' => 'My number'],
['name' => 'mybool', 'type' => 'boolean', 'default' => true, 'label' => 'Option A'],
['name' => 'mylist', 'type' => 'array', 'data' => ['one', 'two', 'three'], 'default' => 'three', 'label' => 'List A'],
['name' => 'mymultilist', 'type' => 'array_multiple', 'data' => ['one', 'two', 'three', 'four'], 'default' => ['one', 'four'], 'label' => 'Multi-select list 2']
];
}
/**
* Main execute command.
*
* @param string $type message type (INFO, WARNING, ERROR)
* @param string $category message category (Config, Action, Application, Security)
* @param string $msg message body
* @return bool true on success, false otherwise
*/
public function execute(string $type, string $category, string $msg): bool
{
// get plugin settings
$config = $this->getConfig();
// get plugin parameter settings
$param1 = $config['parameters']['myparameter1'];
$param2 = $config['parameters']['myparameter2long'];
// do action
$result = false;
if ($param1 === 'XYZ') {
// run some action
$result = $this->someImportantAction($param2, $msg);
// ...etc.
}
return $result;
}
private function someImportantAction($param, $msg)
{
// do some action...
}
}
Bacula configuration plugin example#
In the Bacularis API project directory is a new directory named Plugins
:
[main project dir]/protected/API/Plugins/
If you have installed Bacularis using binary packages, the path can be the following for the Bacularis API plugins:
/usr/share/bacularis/protected/API/Plugins/
In the Plugins
directory please create a new PHP file that will contain
the plugin code. Exmaple:
/usr/share/bacularis/protected/API/Plugins/ExamplePlugin.php
It will contain a PHP class that needs to implement an interface according to
desired plugin type. In this example we use the notification plugin interface
IBacularisBaculaConfigurationPlugin
. The plugin class should extend the
plugin base class BacularisAPIPluginBase
.
<?php
use Bacularis\Common\Modules\IBacularisBaculaConfigurationPlugin;
use Bacularis\API\Modules\BacularisAPIPluginBase;
class ExamplePlugin extends BacularisAPIPluginBase implements IBacularisBaculaConfigurationPlugin
{
/**
* Get plugin name displayed in API panel.
*
* @return string plugin name
*/
public static function getName(): string
{
return 'My example plugin';
}
/**
* Get plugin version.
*
* @return string plugin version
*/
public static function getVersion(): string
{
return '1.0.0';
}
/**
* Get plugin type.
For Bacula configuration plugins it should be 'bacula-configuration'.
*
* @return string plugin type
*/
public static function getType(): string
{
return 'bacula-configuration';
}
/**
* Get plugin configuration parameters.
*
* return array plugin parameters
*/
public static function getParameters(): array
{
return [
['name' => 'pre_create_command', 'type' => 'string', 'default' => '', 'label' => 'Pre-create config command'],
['name' => 'post_create_command', 'type' => 'string', 'default' => '', 'label' => 'Post-create config command'],
['name' => 'write_config_command', 'type' => 'string', 'default' => '', 'label' => 'Write Bacula config command']
];
}
/**
* Pre-config read action.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
*/
public function preConfigRead(?string $component_type, ?string $resource_type, ?string $resource_name): void
{
// do something on pre-config read action
}
/**
* Post-config read action.
* Action is called if the configuration is read successfully.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
* @param array $bconfig Bacula configuration
*/
public function postConfigRead(?string $component_type, ?string $resource_type, ?string $resource_name, array $bconfig = []): void
{
// do something on post-config read action
}
/**
* Pre-config create action.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
* @param array $bconfig Bacula configuration to add
*/
public function preConfigCreate(?string $component_type, ?string $resource_type, ?string $resource_name, array $bconfig = []): void
{
$config = $this->getConfig();
if ($config['parameters']['pre_create_command']) {
$this->exampleAction123(
$config['parameters']['pre_create_command'],
$bconfig
);
}
}
/**
* Post-config save action.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
* @param array $bconfig all Bacula configuration after saving
*/
public function postConfigCreate(?string $component_type, ?string $resource_type, ?string $resource_name, array $bconfig = []): void
{
$config = $this->getConfig();
if ($config['parameters']['post_create_command']) {
$this->exampleAction123(
$config['parameters']['post_create_command'],
$bconfig
);
}
}
/**
* Pre-config update action.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
* @param array $bconfig Bacula configuration to update
*/
public function preConfigUpdate(?string $component_type, ?string $resource_type, ?string $resource_name, array $bconfig = []): void
{
// do something on pre-config update action
}
/**
* Post-config update action.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
* @param array $bconfig all Bacula configuration after updating
*/
public function postConfigUpdate(?string $component_type, ?string $resource_type, ?string $resource_name, array $bconfig = []): void
{
// do something on post-config update action
}
/**
* Pre-config delete action.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
*/
public function preConfigDelete(?string $component_type, ?string $resource_type, ?string $resource_name): void
{
// do something on pre-config delete action
}
/**
* Post-config delete action.
*
* @param string $component_type Bacula component type (dir, sd, fd, bcons)
* @param string $resource_type Bacula resource type (Job, Fileset, Client ...etc.)
* @param string $resource_name Bacula resource name (MyJob, my_client-fd ...etc)
*/
public function postConfigDelete(?string $component_type, ?string $resource_type, ?string $resource_name): void
{
// do something on post-config delete action
}
/**
* Read Bacula config action.
*
* @param string $bconfig Bacula configuration
*/
public function readConfig(string $bconfig): void
{
// do something on read Bacula config action
}
/**
* Write Bacula config action.
*
* @param string $bconfig Bacula configuration
*/
public function writeConfig(string $bconfig): void
{
$config = $this->getConfig();
if ($config['parameters']['write_config_command']) {
$cmd = $config['parameters']['write_config_command'];
$this->exampleAction456(
$cmd,
$bconfig
);
}
}
/**
* Example action 123
*
* @param string $cmd command
* @param array $bconfig Bacula config
*/
private function exampleAction123(string $cmd, array $bconfig): void
{
// do something
}
/**
* Example action 456
*
* @param string $cmd command
* @param string $bconfig Bacula config
*/
private function exampleAction456(string $cmd, string $bconfig): void
{
// do something
}
}
Bacula backup plugin example#
In the Bacularis Common project directory is a new directory named Plugins
:
[main project dir]/protected/Common/Plugins/
If you have installed Bacularis using binary packages, the path can be the following for the Bacularis Common plugins:
/usr/share/bacularis/protected/Common/Plugins/
In the Plugins
directory please create a new PHP file that will contain
the plugin code. Exmaple:
/usr/share/bacularis/protected/Common/Plugins/ExamplePlugin.php
It will contain a PHP class that needs to implement an interface according to
desired plugin type. In this example we use the Bacula backup file daemon
plugin interface IBaculaBackupFileDaemonPlugin
. The plugin class should extend the
plugin base class BacularisCommonPluginBase
.
<?php
namespace Bacularis\Common\Plugins;
use Bacularis\Common\Modules\IBaculaBackupFileDaemonPlugin;
use Bacularis\Common\Modules\BacularisCommonPluginBase;
class ExamplePlugin extends BacularisCommonPluginBase implements IBaculaBackupFileDaemonPlugin
{
private const BACKUP_COMMAND = ['tar', '-cf', '/dev/stdout'];
private const RESTORE_COMMAND = ['tar', '-C', '/', '-xvf', '-'];
/**
* Get plugin name displayed in Web and API panel.
*
* @return string plugin name
*/
public static function getName(): string
{
return 'My example plugin';
}
/**
* Get plugin version.
*
* @return string plugin version
*/
public static function getVersion(): string
{
return '1.0.0';
}
/**
* Get plugin type.
For Bacula configuration plugins it should be 'bacula-configuration'.
*
* @return string plugin type
*/
public static function getType(): string
{
return 'backup';
}
/**
* Get plugin configuration parameters.
*
* return array plugin parameters
*/
public static function getParameters(): array
{
return [
[
'name' => 'backup-path',
'type' => 'string',
'default' => '',
'label' => 'Directory to backup'
]
];
}
/**
* Plugin module initialize method.
*
* @param array $args plugin arguments
*/
public function initialize(array $args): void
{
// here put the initialize actions
}
/**
* Backup command.
*
* @param array $args backup command arguments
* @return bool true on success, false otherwise
*/
public function doBackup(array $args): bool
{
$ret = false;
if (key_exists('backup-path', $args)) {
$cmd = self::BACKUP_COMMAND;
$cmd[] = $args['backup-path'];
$result = $this->execCommand($cmd, true);
$ret = ($result['exitcode'] == 0);
}
return $ret;
}
/**
* Restore command.
*
* @param array $args restore command arguments
* @return bool true on success, false otherwise
*/
public function doRestore(array $args): bool
{
$result = $this->execCommand(self::RESTORE_COMMAND, true);
$ret = ($result['exitcode'] == 0);
return $ret;
}
/**
* Get backup command.
*
* @param array $args script parameters
* @return string backup command
*/
private function getBackupCommand(array $args): string
{
$action = 'command/backup';
$backup_params = [];
$backup_params['plugin-name'] = $args['plugin-name'];
$backup_params['backup-path'] = $args['backup-path'] ?? '';
$cmd = $this->getPluginCommand(
$action,
$backup_params
);
return implode(' ', $cmd);
}
/**
* Get restore command.
*
* @param array $args script parameters
* @return string restore command
*/
private function getRestoreCommand(array $args): string
{
$action = 'command/restore';
$restore_params = [];
$restore_params['plugin-name'] = $args['plugin-name'];
$cmd = $this->getPluginCommand(
$action,
$restore_params
);
return implode(' ', $cmd);
}
/**
* Get plugin command list returned by main fileset plugin command.
* (@see IBaculaBackupPlugin::getPluginCommand);
*
* @param array $args plugin command parameters
* @return array plugin commands
*/
public function getPluginCommands(array $args): array
{
$cmds = [];
if (key_exists('backup-path', $args)) {
$bpipe = $this->getModule('bpipe');
$backup_cmd = $this->getBackupCommand($args);
$restore_cmd = $this->getRestoreCommand($args);
$cmds[] = $bpipe->getPluginCommand(
$args['backup-path'],
$backup_cmd,
$restore_cmd
);
}
return $cmds;
}
/**
* Get plugin restore parameter categories.
* It should return all parameter categories that are used in restore.
*
* @return array plugin parameter categories
*/
public static function getRestoreParameterCategories(): array
{
return [];
}
}
Parameters#
Defined in the getParameters()
method plugin parameters become automatically
available on the web interface. They are ready to configure in auto-generated
HTML form. After saving the form, there is created a new settings for the
plugin.
The getParameters()
method return array of parameters. Each parameter has
defined type
property that determines what type of HTML field the parameter
will be represented. Below you can find descriptions for supported parameters by
type
.
string
- it is a simple input text field. It supports the following properties:
name
- short parameter name
default
- default value
label
- parameter name displayed on the web interface.
string_long
- it is a texarea type text field. It is for storing multi-line parameter values. The line separator is the new line\n
character. It supports the following properties:
name
- short parameter name
default
- default value
label
- parameter name displayed on the web interface.
integer
- it is a simple input text field. It supports the following properties:
name
- short parameter name
default
- default value
label
- parameter name displayed on the web interface.
boolean
- it is a checkbox type field. It supports the following properties:
name
- short parameter name
default
- default boolean value (true/false)
label
- parameter name displayed on the web interface.
array
- it is a drop down list type field with list of values to select. There is possible to select only one value. It supports the following properties:
name
- short parameter name
default
- default value
label
- parameter name displayed on the web interface.
data
- array of available value items
array_multiple
- it is a drop down list type field with list of values to select. There is possible to select multiple values. It supports the following properties:
name
- short parameter name
default
- array of default values
label
- parameter name displayed on the web interface.
data
- array of available value items
The form for this example plugin looks like on the image below.
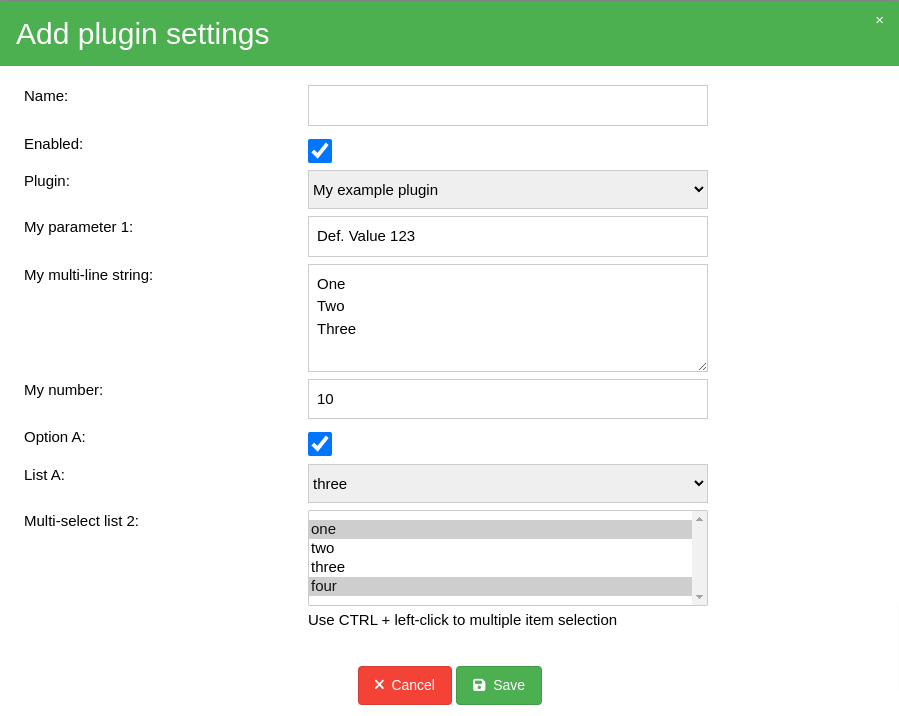